Overview
I bought an Amazon Echo during my last trip to Boise Idaho in US. It is cool product to respond quickly and works so well in acceptable noisy environment. She is a good buddy for my 5 year-old boy to play with and learn English from.
And I want her do more for more automation stuff at home. All my appliance are traditional ones. I bought them 2 years ago when I moved to the new condo. That’s not a long time passed but I don’t even know how technology changes so fast. Every electrical stuff becomes smart even a socket or a plug. That’s a amazing!
With Alexa, I think I can do more to make my home smarter even with the old style appliances.
The first thing comes into my eys is Broadlink universal remote control. It’s actually a converter for WiFi to IrDa and RF signal. Here is the product link. With the app provided by Broadlink, it can learn any IrDA or RF code of the remote controller and build in software virtual remote controller in the app to control the appliances those it can reach. There are 2 apps in Play Store:
But only using Broadlink remote controller is not perfect. You have to pick up your phone and click several times to open the app and corresponding virtual remote controller. This is not what I want.
To integrate Alexa, there are several solutions from time Googling.
- The appliance is born to be smart. And the vendor provides Alexa skills to control the appliance. For example, Philips HUE light, Belkin Wemo and etc.
- Integrate Broadlink into Alexa. There are also many solutions for this, including:
1) Android + Broadlink RM Plugin + Alexa
2) Domoticz/Home Assistant + HA-Bridge + Alexa
3) Domoticz + python plugin + controlicz + Alexa
4) Broadlink native skill + Alexa - Domoticz/Home Assistant + HomeBridge + Siri
Domoticz and Home Assistant are both Home Automation System. There are a lot of people practicing them and post their experience on Internet. (They help me a lot. And this is the time I want to share my experience with others.) And both systems have their own advantages and disadvantages. The reference link 1 is the comparison among 5 popular automation systems.
I choose Domoticz is because it has native support the Synology NAS OS - DSM. Home NAS is in 7*24 hours service. I don’t need to setup extra hardware for the system. With this idea in mind, I practice solution 2)~4) in the item 2. I will consolidate all of them in this post below. The final solution architecture is shown as below:
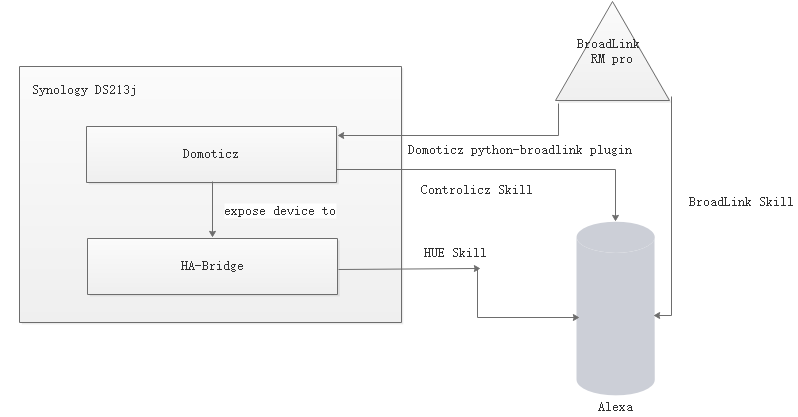
Domoticz into Synology
This is quite simple. Please see the official website - Domoticz for Synology NAS. Download Domoticz for Synology DSM 6.1 with Python Plugin Beta. Python plugin is very important, don’t miss it. It will be used in after chapters.
The file you download is a .spk file. This is a installable package of Synology DSM. And you can install it by clicking “Manual Install” in “Package Center”. After success, it will shown as below:
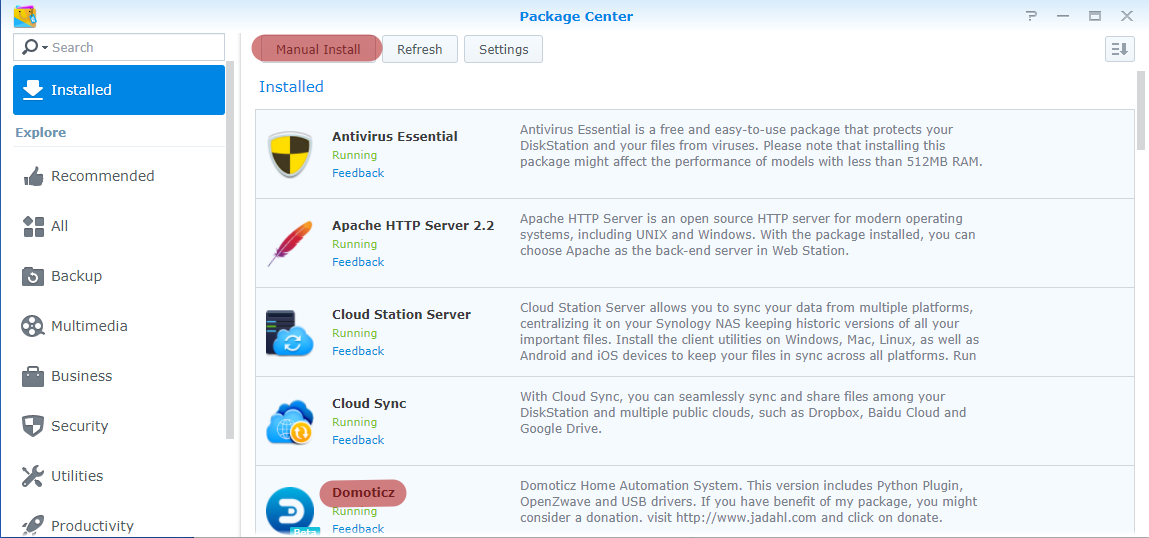
Other Dependency
Domoticz’s plugin system depends on Python3
broadlink-http-rest server depends on Python2
HA-Bridge Server depends on Java8
You’d better to have also “git” installed. That will be much easier to clone GitHub projects.
The 4 packages in Synology package center look like below.
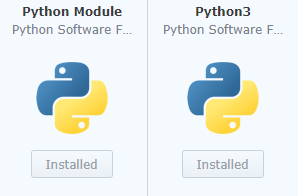
Broadlink into Domoticz
Domoticz Broadlink Python Plugin + Controlicz
This part takes the most issues and I spent several nights fixing the issues. I will have the issue list below.
Domoticz Broadlink plugin and broadlink-http-rest are all based on python-broadlink. So first of all, clone the project python-broadlink from GitHub.
Install python-broadlink
In many posts, people suggest to use pip to install the library of python-broadlink. But I found an issue of doing it this way. python-broadlink once was built based on pycrypto, which is out of support. And then the author switch to pyaes. But I think he didn’t resolve the dependency between python-broadlink and pycrypto. If you install python-broadlink on Synology using pip, it will run into issues of compiling pycrypto. Apparently, Synology DSM doesn’t have compilation environment (GCC and etc.).
My suggested steps are:
- Make sure your python is linked to Python3. (ex. ln -s /usr/local/bin/python3.5 /usr/bin/python)
- git clone python-broadlink && cd python-broadlink
python setup.py install
python -m pip install pyaes
. Because pip3 cannot be installed into Synology DSM, even it’s installed by python3.5 get-pip.py
. The script will also install pip2.
Install Domoticz Python Plugin
Reference link 3 is a Domoticz wiki page provided by the plugin author. The post is mainly worked out based on Windows system, but referring the others chapters, it’s still feasible. The installation steps are:
- Download plugin files from dropbox.
- The plugin folder is in
/usr/local/domoticz/var/plugins/BroadlinkRM2/
. Copy the files to this folderincluding:
- plugin.py -main file
- plugin_send.py
- plugin_http.py
- plugin_http.sh
Be noted: the plugin folder is not /usr/local/domoticz/plugins/BroadlinkRM2/
, although there is an example folder in that directory. But when I put plugin files in that directory, the plugin won’t be loaded correctly.
- Stop and Run Domoticz in Package Center of Synology DSM. And do this every time you update the plugin python file.
So far, the installation finished. You should be able to see the Broalink hardware type in the pull-down list in Setup->Hardware.
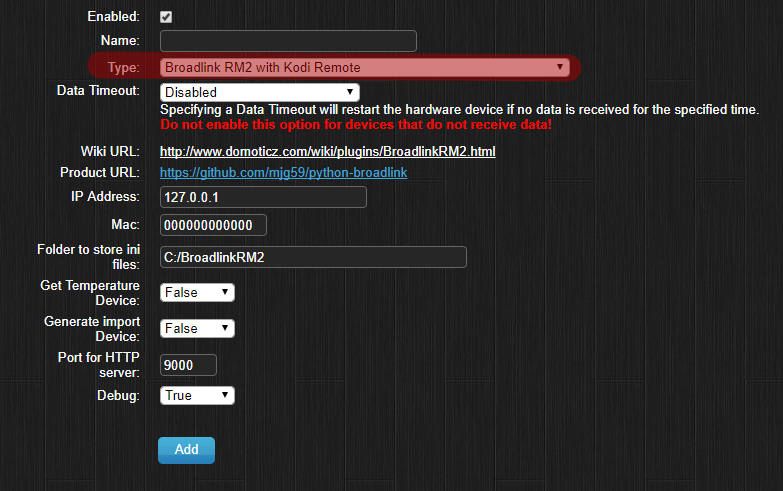
Create Broadlink Hardware
Following the author’s steps, you should be able to create broadlink hardware in Domoticz web page and discover broadlink devices.
To create the hardware as below:
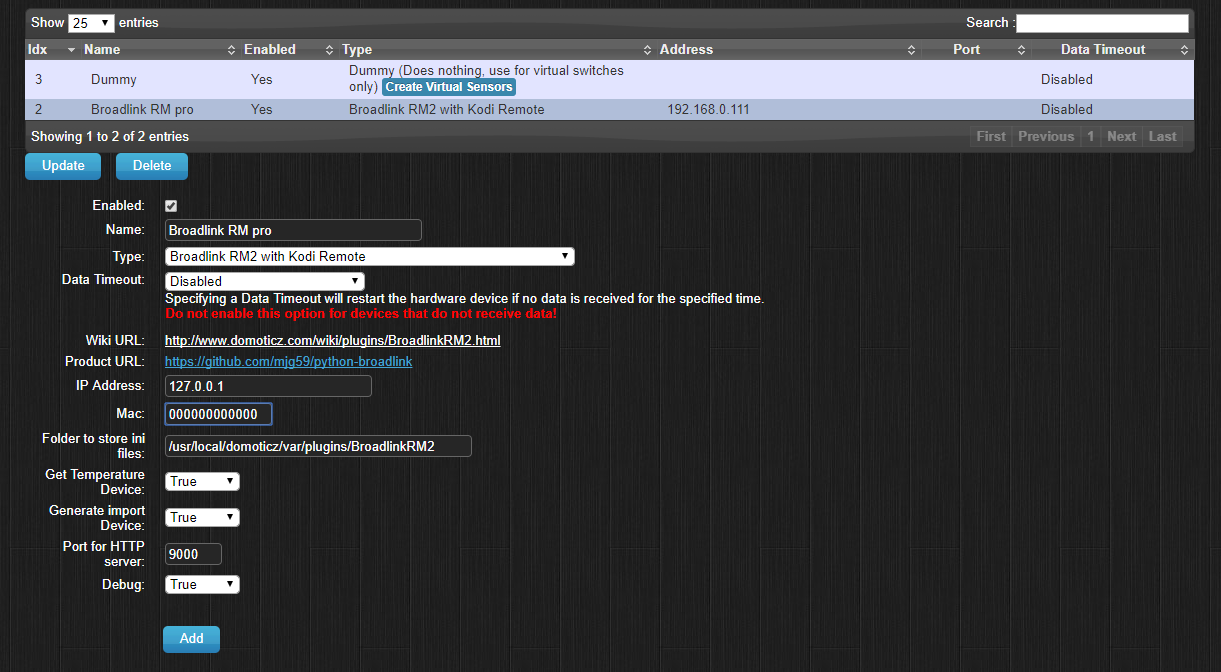
Import Devices from E-Control App导入易控(e-control)设备
Refer to reference link 3 for detailed description. It’s in User Guide->Inside Domoticz->xxx - import chapter. Author may not work with multi-byte language, such as Chinese. If there are Chinese characters in the imported json* files, the plugin will crash. See issue 3 for solution.
Another point should be noted, when WebStart is triggered, Domoticz will setup a tiny web service running on port 9000 to handle the files uploaded from mobile devices. Make sure you configure the Synology firewall correctly.
Controlicz
This seems to be a new service. I don’t see people mention it when I’m reading through Google results. I happened to see it in Alexa skill page. Using Controlicz, you don’t need to simulate your appliances as Philips HUI light using HA-Bridge. But it has its own pros and cons with Broadlink remote controller. There is a dedicated section comparing Controlicz and HA-Bridge.
To use Controlicz:
- Register Controlicz using your Alexa’s credential (email and password)
- Enable skill and say “discover device” to Alexa.
- All done
Be noted: Controlicz asks for:
- Domoticz service should be exposed to external access. That means you need to map port and DDNS. Because I’m using Synology, that’s not a problem to me.
- Controlicz asks Domoticz running on HTTPS protocol. That means you have to enable the Domoticz HTTPS port and corresponding Firewall configurations.
Issue List
So far, if you are lucky enough to succeed in all above steps, you can say “Turn on the TV” to Alexa. The TV should respond to you quickly and correctly. If unfortunately it doesn’t, don’t worry. Check the issue list to see if it helps.
Import Error “broadlink”
You have installed python-broadlink already, and you can import it from Python REPL command line. Why is the error still reported? The direct cause is the PYTHONPATH is incorrect. But when I check the code in plugin.py, I see the process of PYTHONPATH as here:
if sys.platform.startswith('linux'):
# linux specific code here
# doesn't work even if set dist-packages => site-packages
sys.path.append(os.path.dirname(os.__file__) + '/dist-packages')
elif sys.platform.startswith('darwin'):
# mac
sys.path.append(os.path.dirname(os.__file__) + '/site-packages')
elif sys.platform.startswith('win32'):
# win specific
sys.path.append(os.path.dirname(os.__file__) + '\site-packages')
The path to broadlink library is /usr/local/lib/python3.5/site-packages/broadlink-0.5-py3.5.egg/broadlink/
. Even when I correct the “dist-package” to “site-packages” in above code snippet, it still cannot work. However, the solution is quite simple. Just copy broadlink to the plugins folder.
cp -r /usr/local/lib/python3.5/site-packages/broadlink-0.5-py3.5.egg/broadlink/ /usr/local/domoticz/var/plugins/BroadlinkRM2/
Error Connecting to Broadlink device
There are 2 GitHub issue link for reference:
# broadlink/__init__.py
160 def encrypt_pyaes(self, payload):
161 aes = pyaes.AESModeOfOperationCBC(self.key, iv = bytes(self.iv))
162 return "".join([aes.encrypt(bytes(payload[i:i+16])) for i in range(0, len(payload), 16)]) # <==== Error is here
bytes in python3 returns class bytes, whereas in python2, it returns plain string. See here for experiments:
Python2
>>> type(bytes([1,2,3]))
<type 'str'>
Python3
>>> type(bytes([1,2,3]))
<class 'bytes'>
>>> "".join([bytes([1,2]),bytes([2,3])]) <==== You cannot cat 2 bytes class objects as string
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: sequence item 0: expected str instance, bytes found
>>> b''.join([bytes([1,2]),bytes([2,3])]) <==== This is the good way
b'\x01\x02\x02\x03'
The correct way to concatenating is to use binary string。
Chinese characters in Domoticz imported files
In Python3, if a file has Chinese character, you cannot read files and decode strings as below:
with open('jsonFile') as f:
textStr = f.read() <=== Python3 will complain about the codec error here.
textStr.decode('utf8')
The correct way is:
with open('jsonFile', encoding='utf8'):
textStr = f.read() <=== Everything here has already been unicode.
Update all lines of opening the imported json* files. And one more thing you need to pay attention to, which is for ConfigParser. You can update the encoding as below for them.
config = configparser.ConfigParser()
config.read(path, encoding='utf8')
Try it, it should do with no problems, aren’t you?
Domoticz + broadlink-http-rest + HA-Bridge
With HA-Bridge, you don’t need Domoticz Python plugin. You can create dummy hardware in Domoticz web page as below picture.
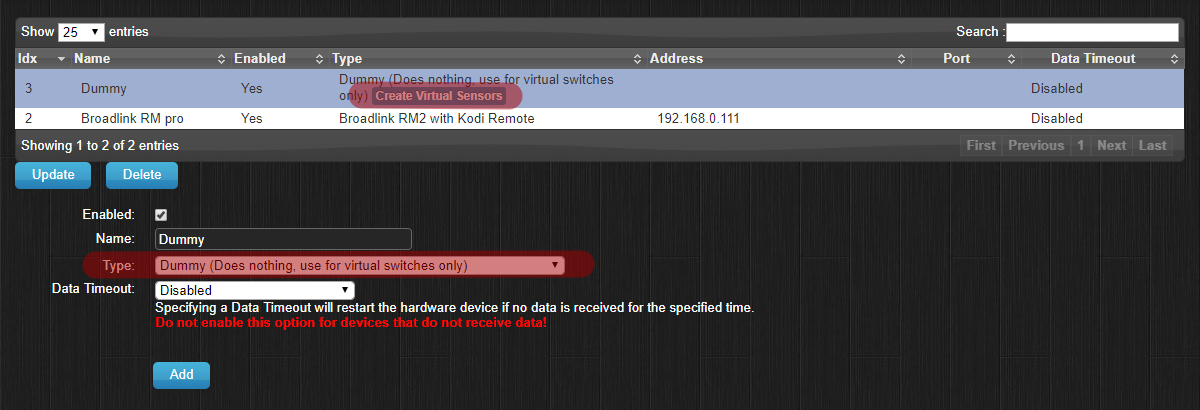
Install HA-Bridge
The whole idea of HA-Bridge is to simulate any device as Philips HUE light, which has native support in Alexa. And HA-Bridge can import all Domoticz devices easily. The cons is it can only support open/close and dim settings like you are operating a HUE light.
HA-Bridge is a jar package works with Java 8. And we need to run it in Synology background as a service. Refer to the post here -Run as a service on Synology for running it as a service.
The command line is nohup java -jar -Dserver.port=8085 /ha-bridge-4.5.6.jar &
. Put it in Synology Scheduled Tasks, and then you don’t need to worry about the reboot.
Install broadlink-http-rest
Clone the project from GitHub - broadlink-http-rest. Just as HA-Bridge, it also needs to be in background as service. Otherwise, it will quite as soon as SSH connection is cut.The command line is nohup python broadlink-http-rest/server.py &
. Be noted, make sure you are using “nohup”, otherwise it will still quite as SSH is disconnected even if you specify the “&”
Firewall & Scheduled Tasks
Configure the firewall:
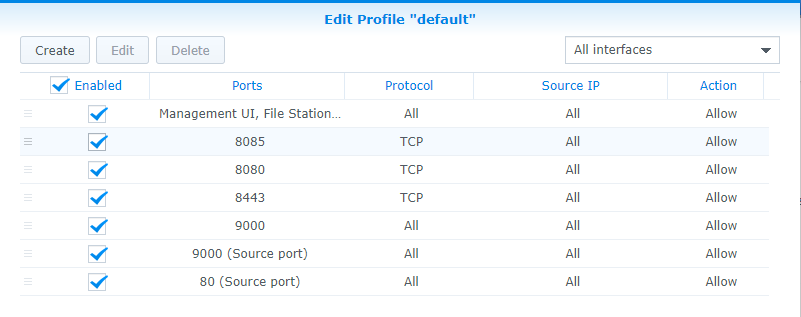
- 8085: HA-Bridge server
- 8080: broadlink-http-rest server
- 8443: Domoticz HTTPS service
- 9000: Domoticz Broadlink web service
Also the scheduled tasks:

Broadlink into Domoticz
The steps are:
- In Setup->Hardware, create a Dummy Hardware
- In the same page and in the created item, click “Create Virtual Sensors”
- In Switches tab, find the virtual sensor you just created, click Edit and configure “On Action” and “Off Action”.
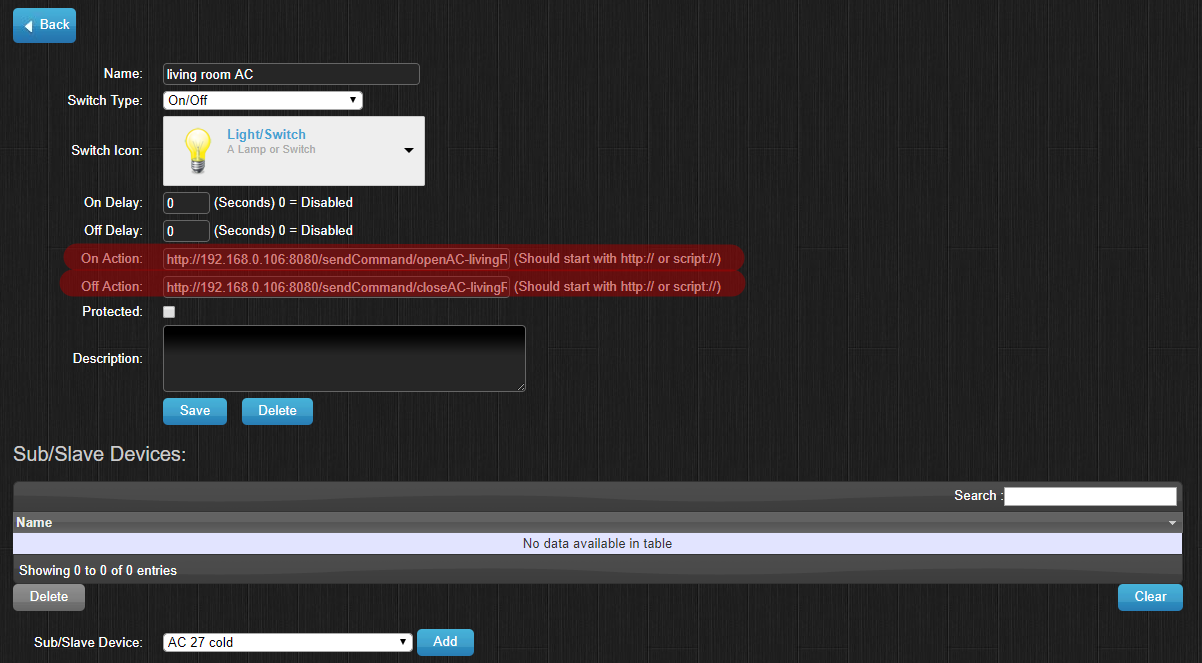
Domoticz into HA-Bridge
Please refer to reference link 2. From the chapter of Configuring HA bridge, it starts to describe how to configure HA-Bridge to import devices from Domoticz web service.
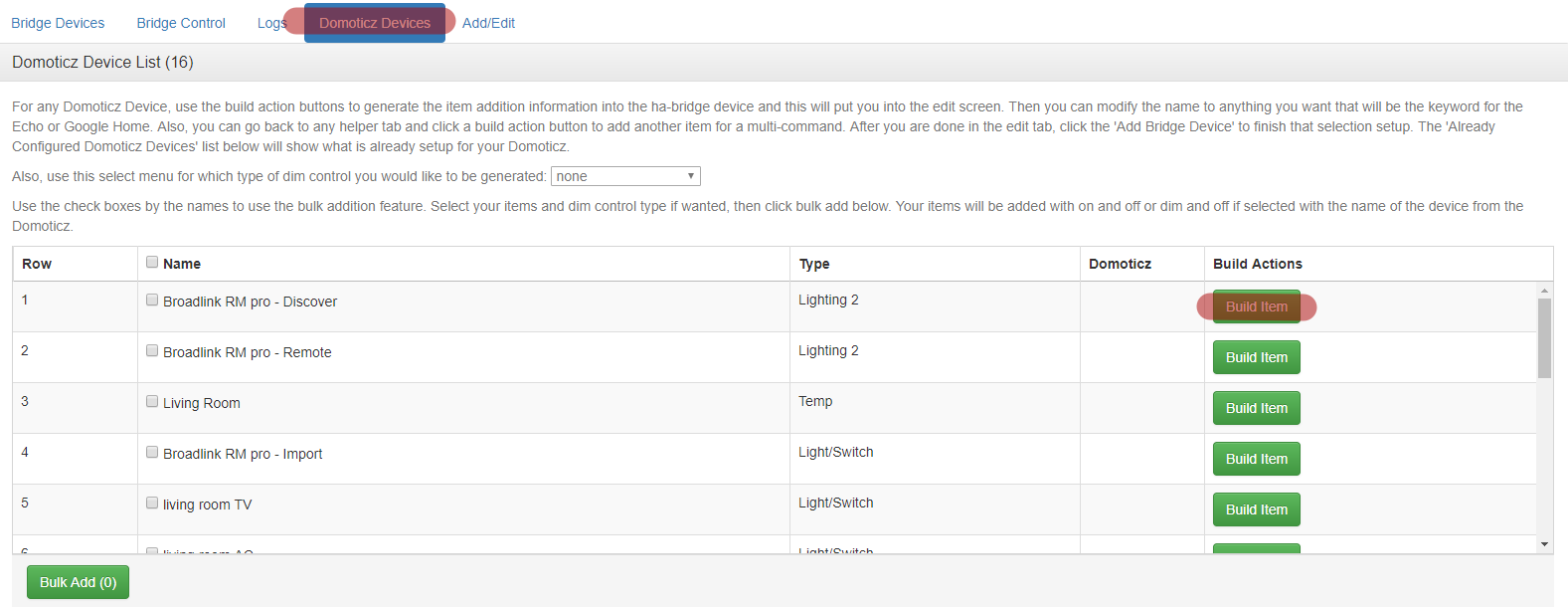
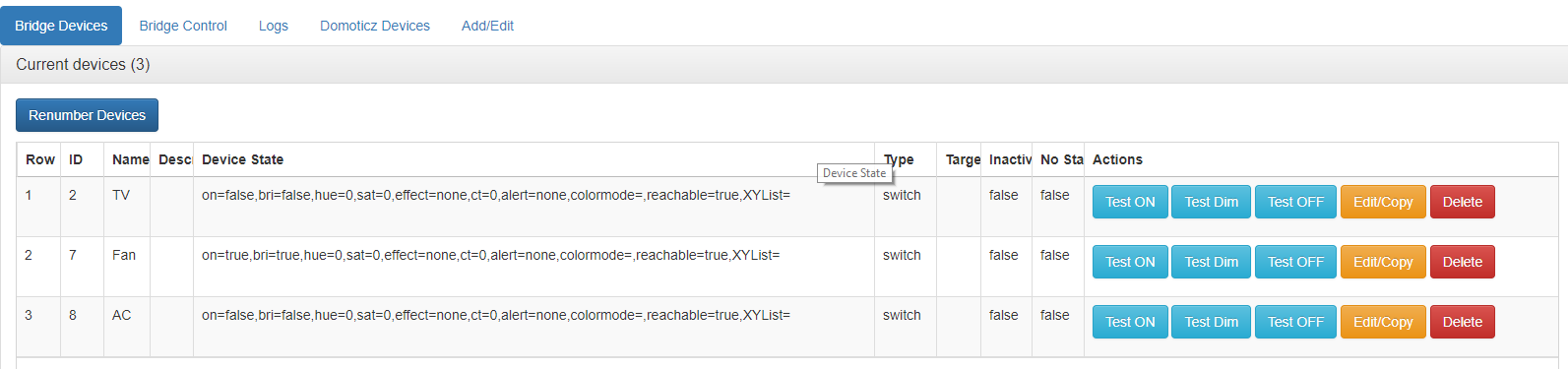
HA-Bridge vs. Controlicz
General Smart Home
Controlicz can integrate all type of devices into Alexa. For example, if Alexa doesn’t have native support of this smart home device, you can build up a Domoticz server in your home and integrate it into Alexa by Controlicz. According to the description in Controlicz official site, it doesn’t only support on/off switches, but also other normal appliance like TV, AC and etc. And Domoticz apparently support a lot more devices than Alexa does.
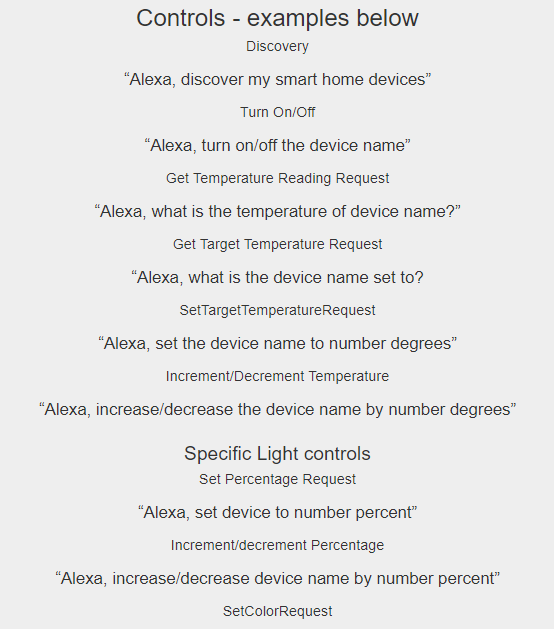
Broadlink Integration
To integrate Broadlink, these 2 have their own pros and cons.
Broadlink is a universal remote controller. So every devices on Broadlink is a push button device. Each one only has one action, which is being triggered. This is even weaker than the on/off switch, which at lease has 2 actions - on or off.
For example, there is a button on Fan’s remote controller. Pushing it once, it will turn on the fan and twice will turn off it. Through Controlicz, you have to say “Turn on the Fan” to Alexa to turn on the fan and you have to say the same sentence to close it. Is it weird?
If you are using HA-Bridge, you can say “Turn on …” to turn on and “Turn off …” to turn off. Is it better?
The biggest benefit of using Controlicz to integrate Domoticz is it can import all devices pre-defined in E-Control app. It can fully make use of the E-Control’s GUI. You don’t need to add virtual sensors one by one manually.
Broadlink Native Skill + IHC
After bunch of work above, I happened to see there is a native support broadlink Alexa skill called “broadlink”. It should work with the latest version of IHC app. The bad news is both the skill and the app have very low review scores.
- IHC playstore 2.2 stars
- Alexa Broadlink Skill 2.3 stars
According to the official examples, it can only support TV. And as my own experiments, when I added a TV and an AC, Alexa can only discover the TV and no AC.
Reference Links
- 5 open source home automation tools
- Alex - Domoticz
- Domoticz Wiki Plugins/BroadlinkRM2
- #原创新人# 群晖安装broadlink-http-rest代替RMBridge_生活记录_什么值得买